In The Mother of Skill post, we talked about the power of actively reading code to improve your skills. In this article, we will kick off the Code Reading series. In each Code Reading episode, we will actively read a public code base in order to work on a particular area of our engineering game.
One thing to remember is that “active reading” requires you to engage with the code. Get in there and pull the code apart, copy it, modify it. Get your hands dirty and break things, then figure out how to get them working again.
For this first code reading, let’s take a look at this repository on Github for a Tic-Tac-Toe web application:
https://github.com/jasonrobertfox/tictactoe
In Part 1 of Code Reading #1, we will give an overview of this code repository as well as what we want to get out of “actively reading” it. There are several skills we can practice with this project, so Code Reading #1 will have multiple parts.
Why This Project?
This application allows a user to play a game of tic-tac-toe against the computer. There is a web UI that the user interacts with in order to select which move he wants to make. The computer uses a min-max algorithm to guide its moves.
This is a good project for several reasons. Back in The Mother of Skill post, we talked about the idea of simplifying the playing field when you want to focus on improving a particular area of your engineering game. This project lends itself well to that concept.
For one, most of us already know how tic-tac-toe works. So there is no need to spend time learning how to use the software.
Furthermore, this application happens to have a clean web interface. The author of the project has kindly provided a link to a deployed version of the application. We can click over and quickly begin familiarizing ourselves with it.
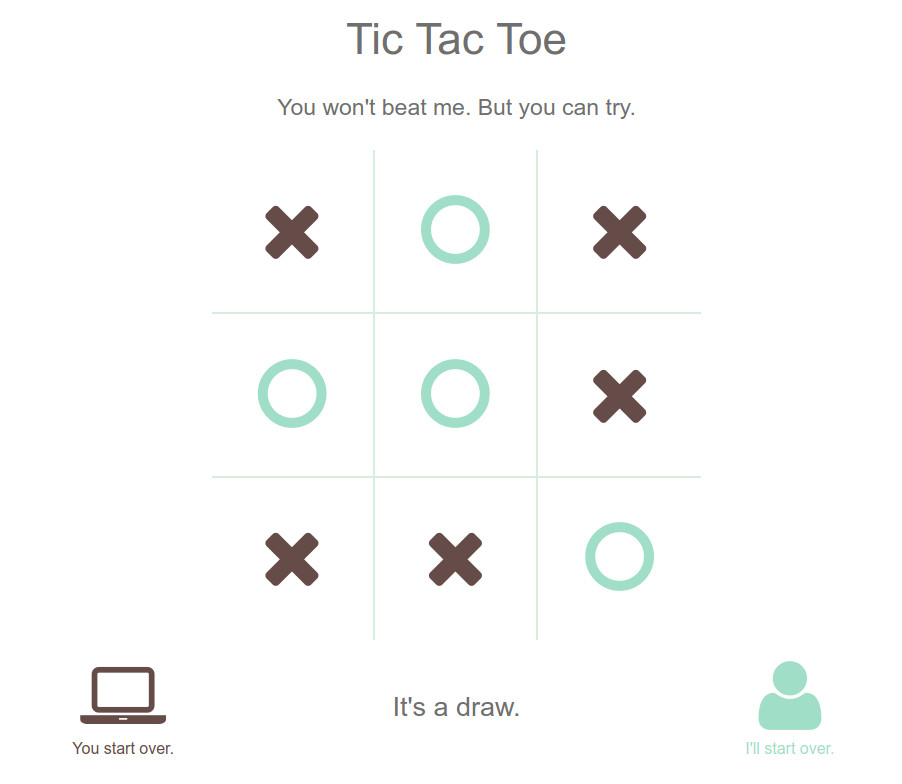
Another reason this project is a good candidate for active reading is that the code base is not dense. The project has 63 files and 28 folders as of this current writing. On a machine running Linux, you should have access to the tree
tool on the command line. Clone or download the repository, then run the tree command on the tictactoe file. You will see that the folder structure is not terribly nested.
tree tictactoe/
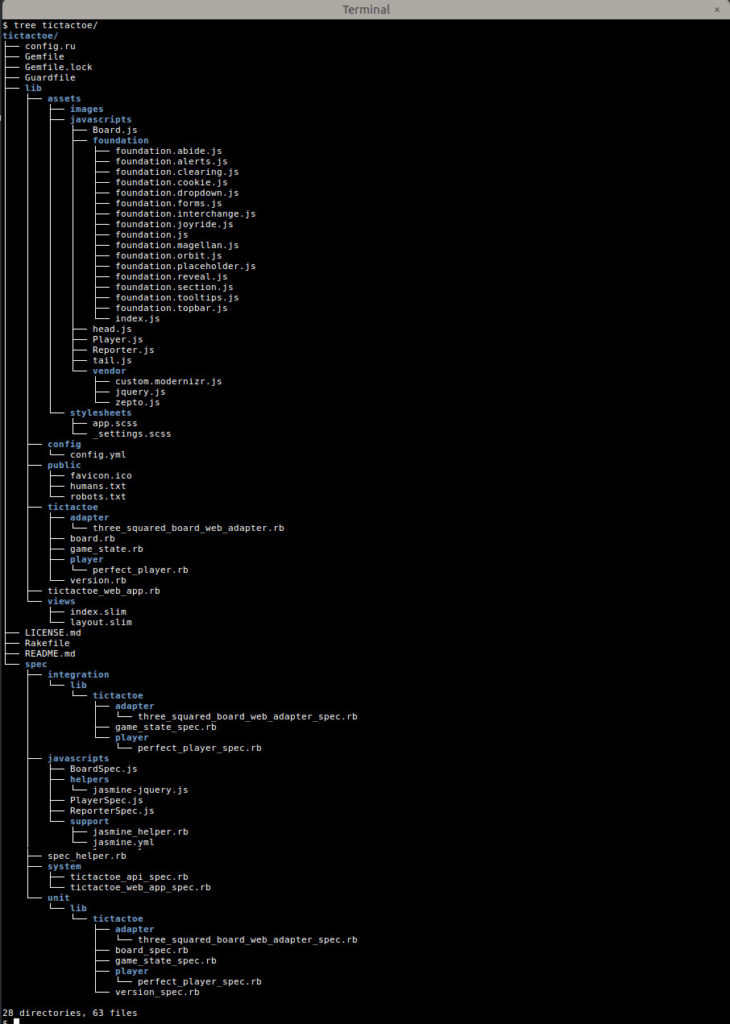
Aside from the benefits listed above, this project can also be used as a tool to practice a variety of skills. For example, you can practice:
- installing and standing up an application from the source code
- containerizing an application
- updating and modifying a technology stack
- breaking a monolithic application into a collection of simpler applications
- adding new features to an existing application
We will tackle each of those areas using this project throughout the various posts for Code Reading #1.
Tech Stack
This application has not been updated in many years, so the tech stack has become dated. The game is built with version 2.0.0 of the Ruby language.
It includes two API endpoints built with a web framework called Sinatra, as well as a suite of tests. The web UI is built using the Foundation front-end framework.
One interesting note about this application is that it is based on a template application called sinatra-boilerplate. In the spirit of simplifying the playing field, we will start our exploration with the sinatra-boilerplate application before adding on the game-specific layer of code.
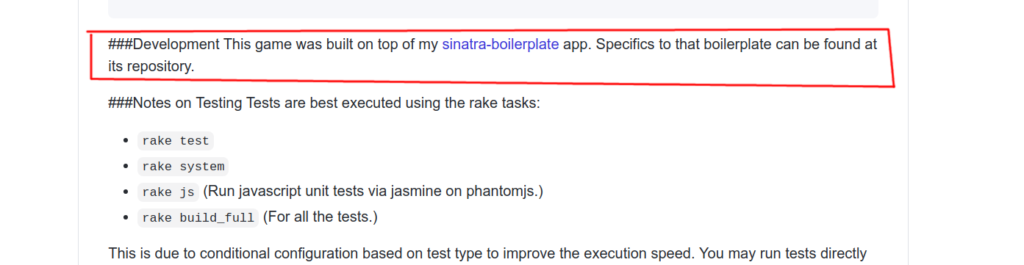
Goals
To start, let’s focus on standing up an application from source code and containerization. These are useful skills because whenever you join an ongoing project, one of your first steps is setting up your development environment. That process includes installing the project and all of its dependencies so you can run it on whatever setup you want to develop with.
Baby Steps
As mentioned earlier, this application is based on a template called the sinatra-boilerplate. Since this tech stack is a bit dated, it is possible we will run into code library compatibility issues. In anticipation of this, let’s not only start with the sinatra-boilerplate (instead of the tic-tac-toe application), but let’s slice it back to a tiny unit of functionality. Let’s cut out the UI and the tests for now and focus on getting a web server with one route working.
Definition of Done
That being said, this first mini session will be done once we can:
- Start up a basic Sinatra application in a Docker container, then successfully hit the
/
route.- The application should run on Ruby 2.0.0
- Hitting
/
should return a response with the text “Hello, Tic Tac Toe!”
Build a Ruby 2.0.0 Image
We will assume that you already have Docker installed on your development machine. The first tiny slice of work will be to create a Docker image around a basic Ruby 2.0.0 application.
Create a folder on your development machine to contain the Ruby code and Dockerfile for this Ruby test.

Open the Ruby file and write a simple line of Ruby code. In the example below, we just output the string "Ruby is running"
.

Now for the Dockerfile. Go to the Ruby section of Dockerhub and click on the Tags tab. Type 2.0.0 in the search box.
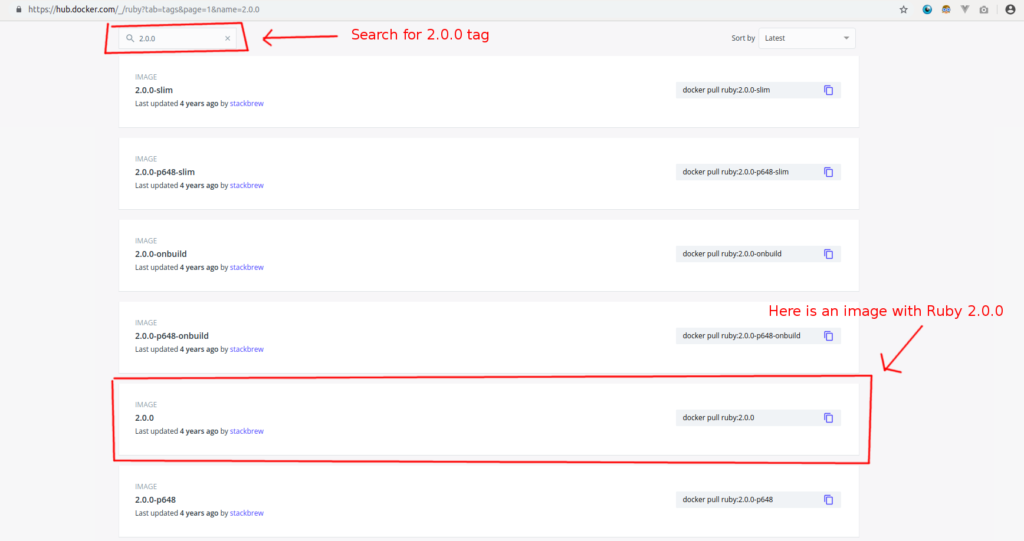
As you can see, there is an image available that already has Ruby 2.0.0 built into it. So when we build our Dockerfile, we can base it on that Ruby 2.0.0 image.
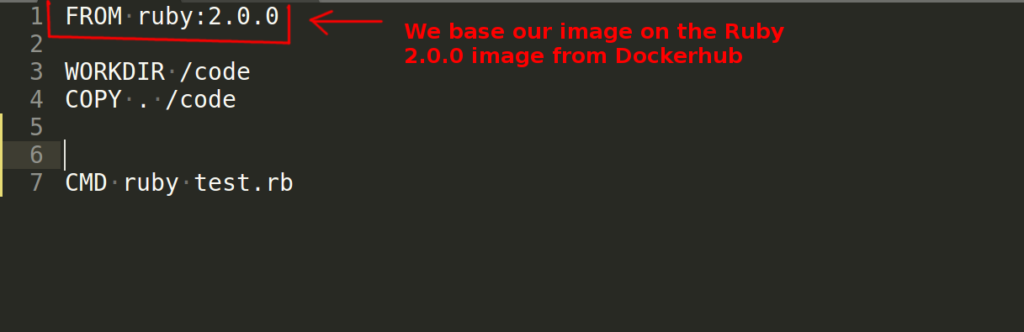
Other than that, we just need to copy in our little Ruby program and then add a command to run it
Now, let’s see if we can successfully build this image. Run the following command from the root of your test Ruby project:
docker build --tag <name of tag> .
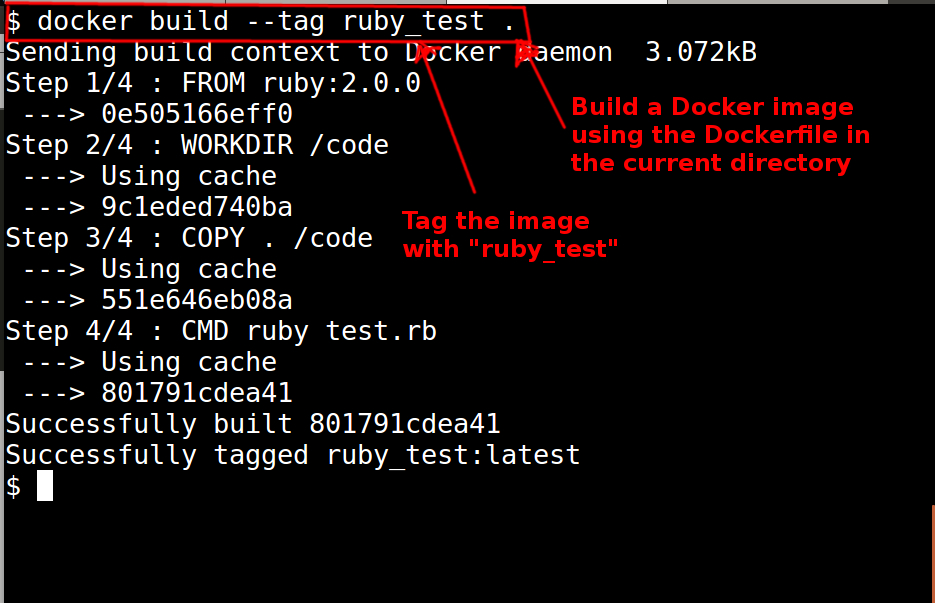
Next, we just need to run a container from this image to see that the program runs. Type the following command to run a container:
docker run <name of tag>
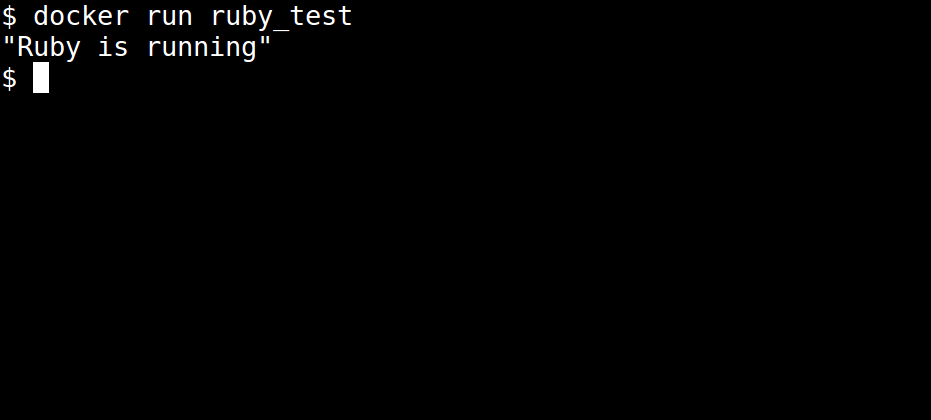
Great – it ran smoothly. That takes care of the first bullet point in our definition of done:
- Start up a basic Sinatra application in a Docker container, then successfully hit the
/
route.The application should run on Ruby 2.0.0[DONE]
- Hitting
/
should return a response with the text “Hello, Tic Tac Toe!”
Add a Compatible Version of Sinatra to the Docker Image
Now that we have successfully gotten a container based on Ruby 2.0.0 running, we can work on adding sinatra to the image.
We need to add a Gemfile to install the sinatra gem. Let’s take a look at the Gemfile from the sinatra-boilerplate project:
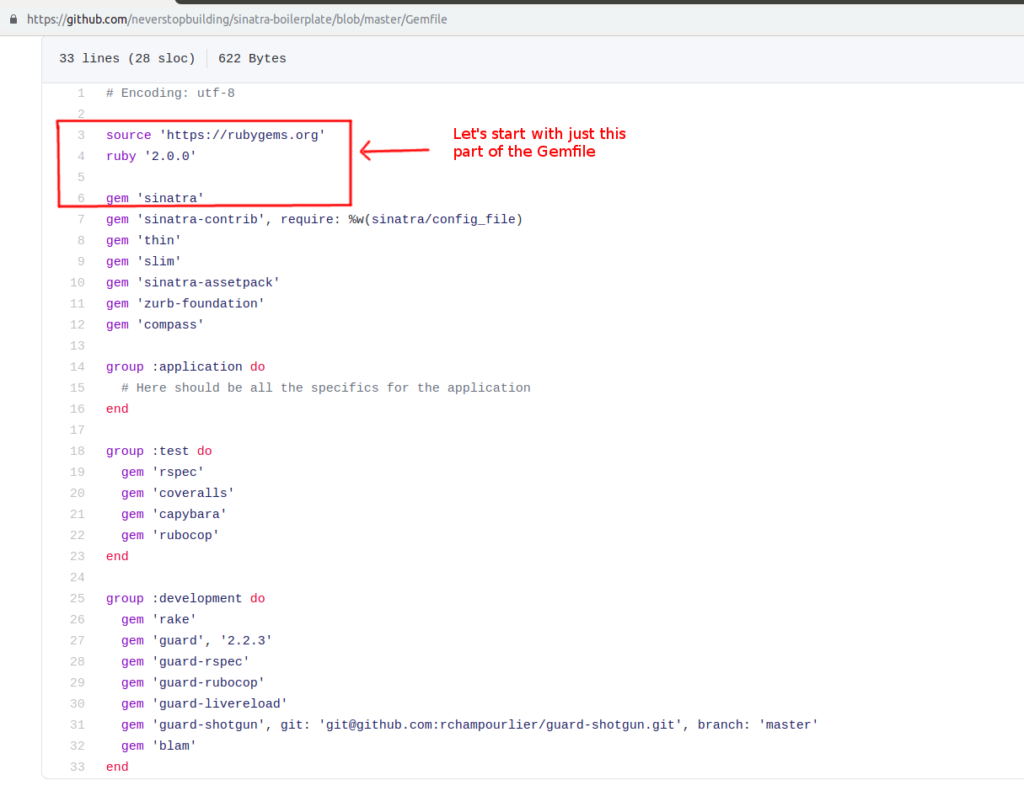
Once again, to simplify the playing field let’s just install the sinatra gem. If we run into dependency conflicts, it will be easier to troubleshoot them if we don’t have to think about all of the gems at the same time. Here’s our Gemfile
:
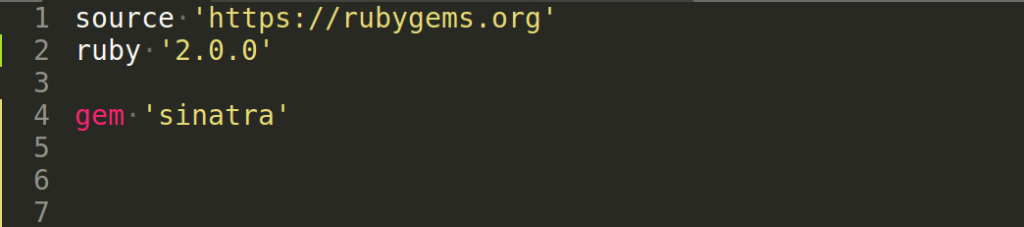
Now that we have introduced a gem and a Gemfile, we will need the Bundler CLI to install that gem and its dependencies. It turns out that the Bundler CLI is already available in the ruby:2.0.0 base image we are using in our Dockerfile.
If you want to do a quick check, take the Dockerfile
we started building when we tested out the ruby application above and run the bundle --version
command: RUN bundle --version
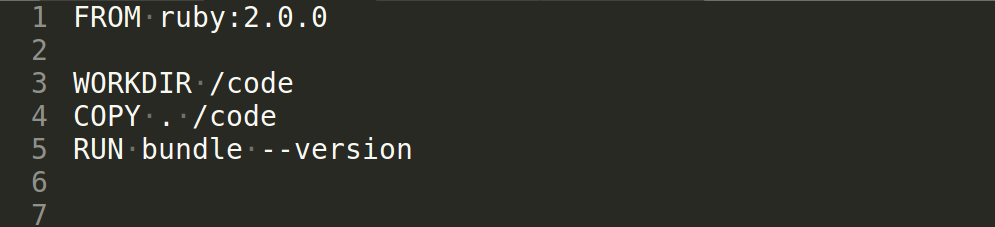
When you build the image, see if an actual version of Bundler is returned.

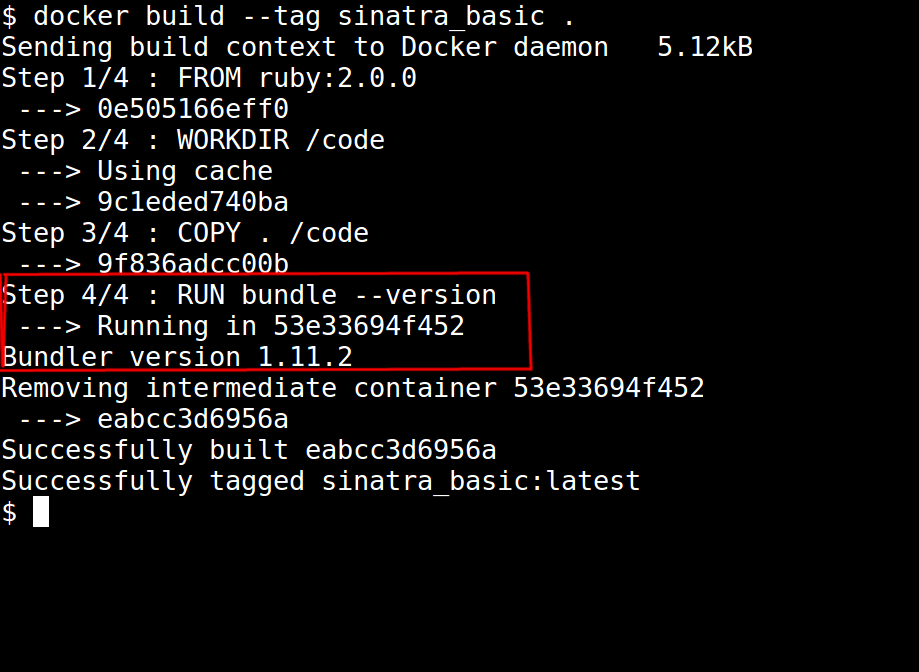
We got a valid version response, so we know we’ll be able to run the Bundler CLI in the container. You can remove this Bundler version test line from the Dockerfile.
Next, go ahead and add the following line to the Dockerfile: RUN bundle install

Let’s build the image now to see if Bundler is able to successfully install the sinatra gem.
Sinatra Compatibility Error
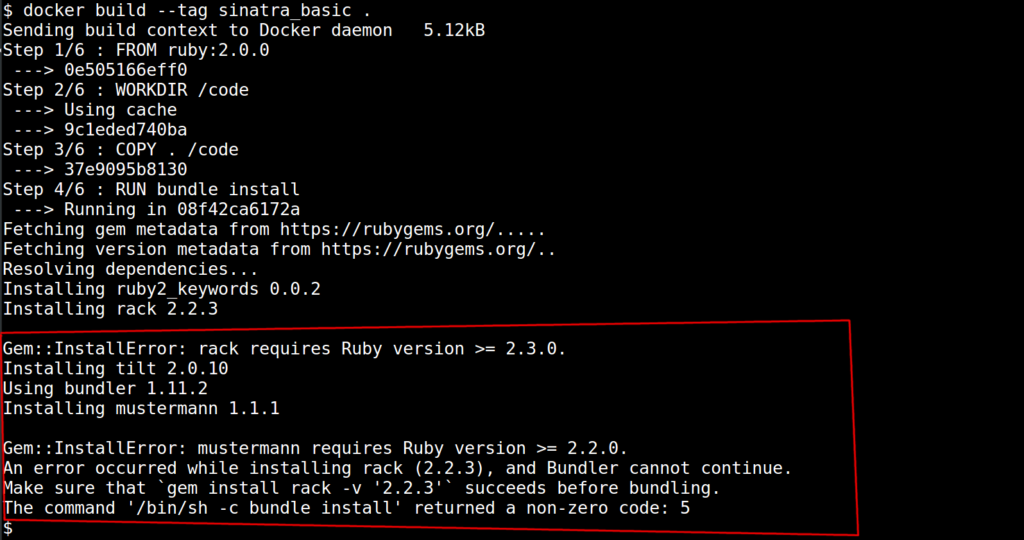
There is an error installing the sinatra gem. Based on the error message, it looks like some of the dependencies for the sinatra gem are incompatible with Ruby version 2.0.0.
The error message does not indicate which version of sinatra Bundler attempted to install. If we examine our Gemfile again, we’ll see that there is no version of sinatra specified. We need to pin sinatra to a version we know will be compatible with Ruby 2.0.0.
Which Version of Sinatra Works with Ruby 2.0.0?
How do you know which version of the sinatra gem works with Ruby 2.0.0? You will have to do a little research. You can always try running search engine queries to pull up an article or forum post with this information.
Another option is to go to the rubygems.org page for the sinatra gem.
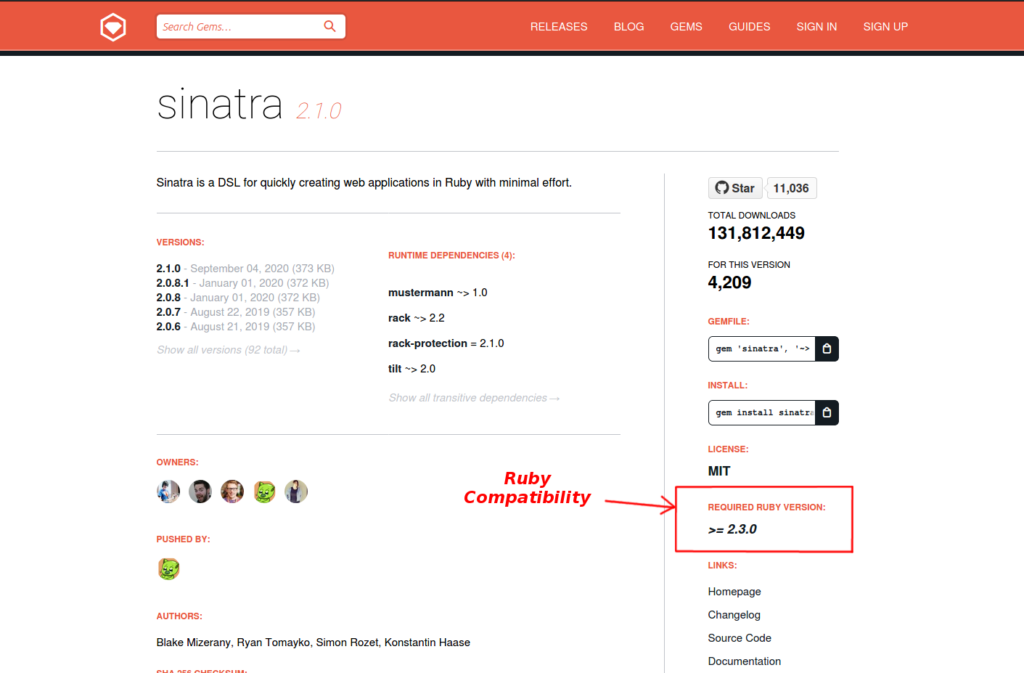
If you look in the column on the right-hand side of the page, you will see a section entitled “Required Ruby Version.” Determining the version of sinatra that is compatible with Ruby 2.0.0 will be a matter of looking through the rubygems.org pages for previous versions of sinatra. If you click on the “Show all versions…” link, you will see a list of the different versions of sinatra that are hosted on rubygems.org.
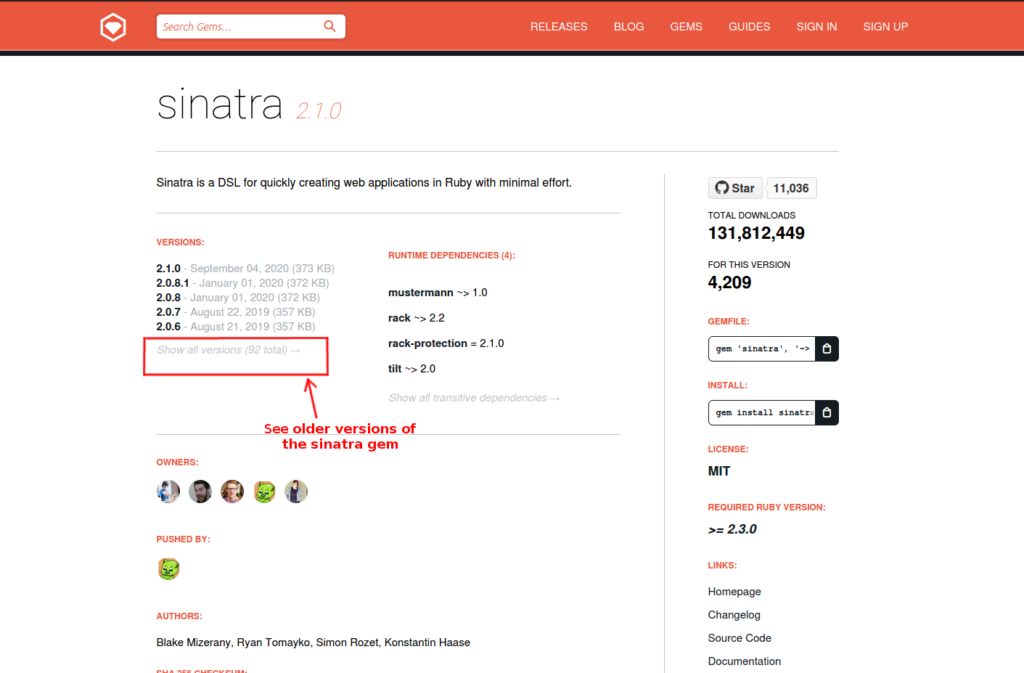
As you click through the versions of sinatra, you will notice that version 2.0.0.beta1 of sinatra requires a version of Ruby later than or equal to 2.2.0. This version of Ruby is not compatible with our application, so let’s go back a version.
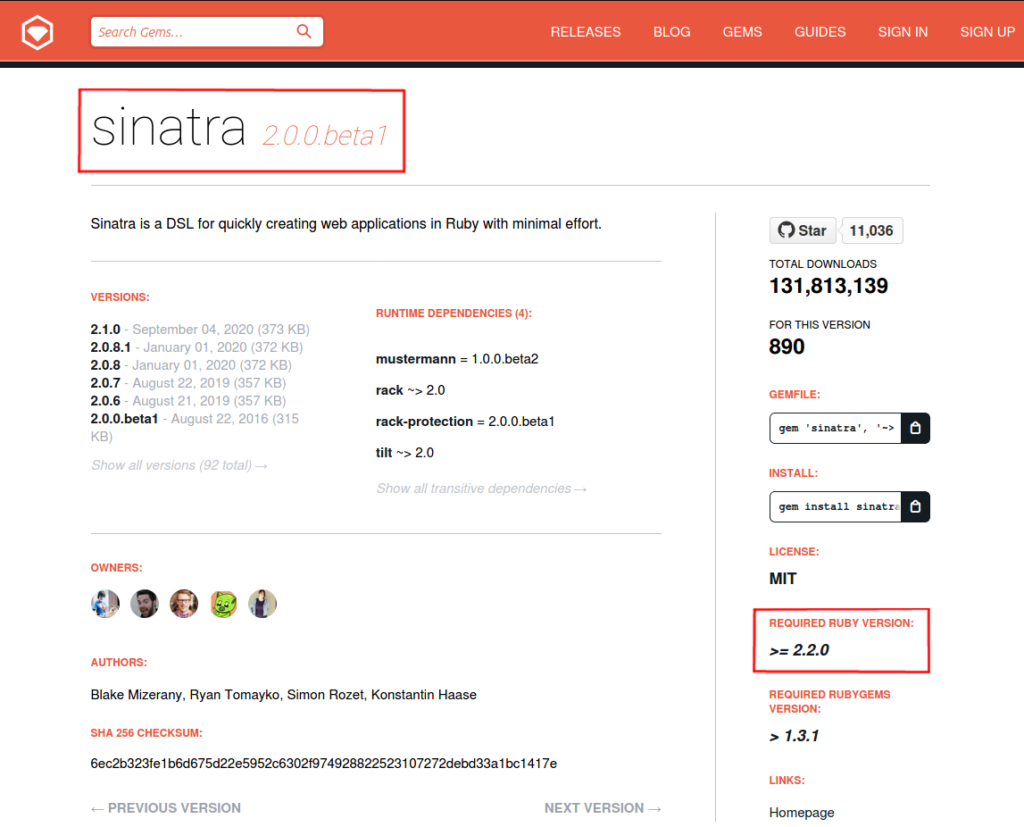
The version of sinatra that came right before version 2.0.0.beta1 is version 1.4.8. Version 1.4.8 of sinatra requires a version of Ruby later than or equal to 0.
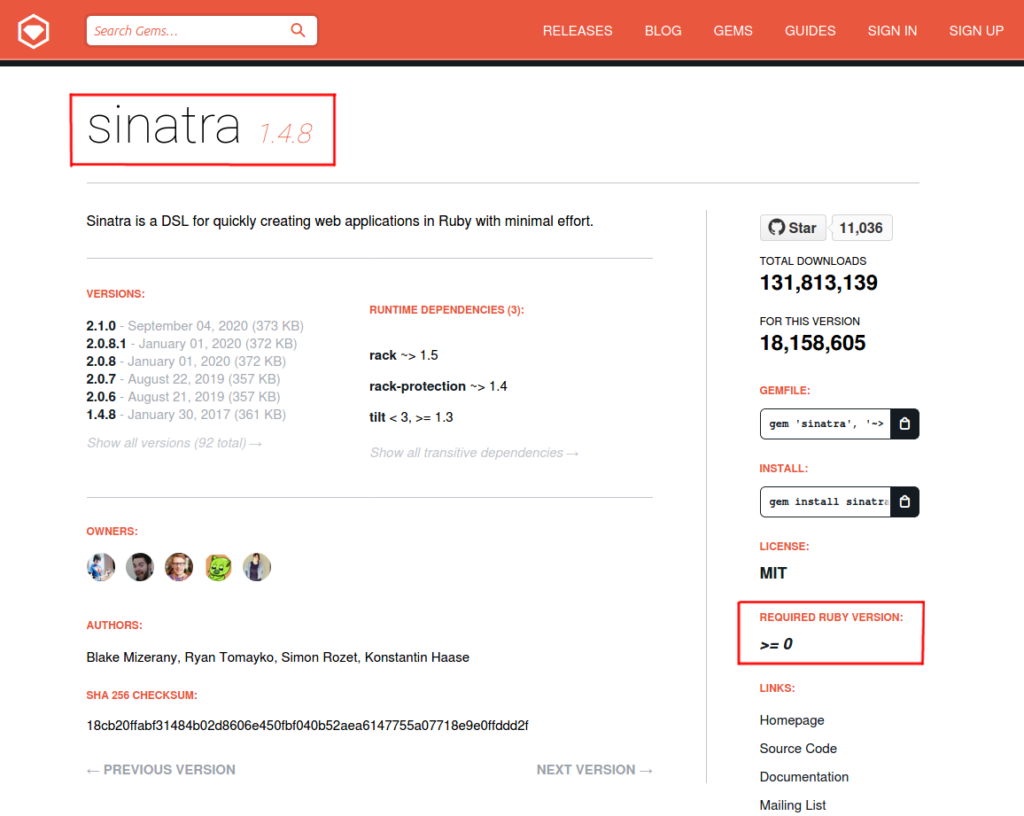
In fact, if you look through versions of sinatra older than 1.4.8, you will see that the Ruby requirement is either listed as NONE
, >= 0
, or >= 0.0.0
. So theoretically, any version of sinatra from 1.4.8 or older should work with Ruby 2.0.0.
We need to test our theoretical finding that any version of sinatra from 1.4.8 or older should work with Ruby 2.0.0. If you test pinning the sinatra version to various gems within the 1.x.x MAJOR version range, it appears that versions from 1.1.x and later are compatible.
Based on this research, we decided to set sinatra’s version to the highest released gem >= 1.1 and < 2.0.
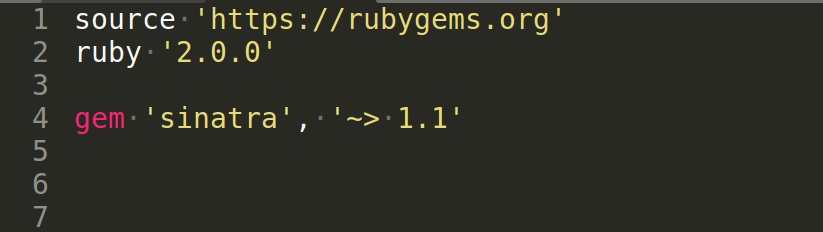
Go ahead and try building the image again to see if sinatra is successfully installed this time.
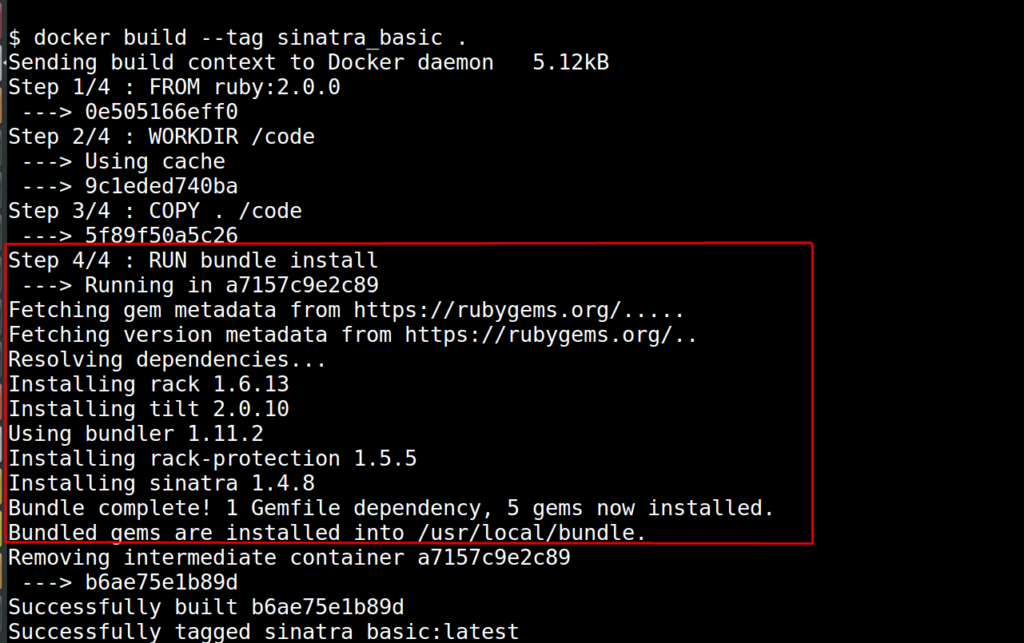
Awesome! We were able to install a version of sinatra that works with Ruby 2.0.0 on our Docker image.
Create a Basic Sinatra Application
Now that we successfully installed the sinatra gem, let’s change our test.rb
file and turn it into a basic Sinatra application. Based on our definition of done, let’s create one handler for the /
route. When we hit this route, the application should return the string: “Hello, Tic Tac Toe!”:
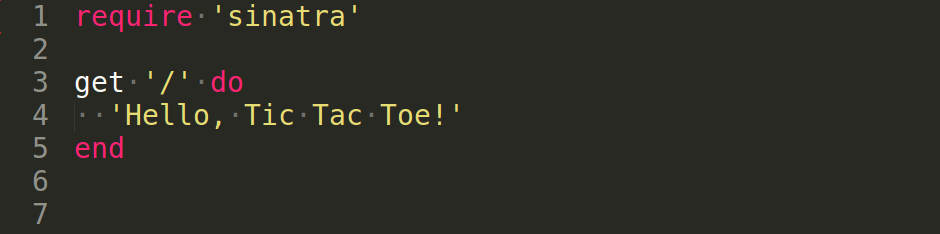
At this point, we should have the following files in our project:
- Gemfile
- Dockerfile
- test.rb
In order to run this Sinatra application, we will need one more file: config.ru
. As you may have noticed when we installed the sinatra gem just now, Sinatra has a dependency on the rack gem. In order to run Sinatra applications, you actually directly run a command from rack called rackup
. But before we run that command, we need to specify the config.ru
file so the rackup command knows what to do.
If you go to the “Getting Started” document for the Sinatra project and scroll down to the section “Using a Classic Style Application with a config.ru,” you will see an example we can follow to set up the config.ru
file for our application. Here’s our config.ru
file:
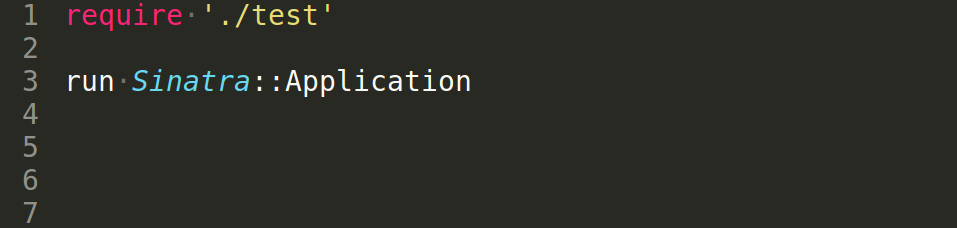
Now that we have the config.ru
set up, there are only two remaining steps. First, we need to make sure the Dockerfile exposes the proper port so we can connect to the application in the Docker container from the world outside of the container (i.e. from our development machine). By default, Sinatra applications listen on port 4567, so we will expose that port.

Next, we need to run the rackup
command from the rack gem in order to start the Sinatra application. This command will seek out the the config.ru
file in whatever directory it is run within:
bundle exec rackup --host 0.0.0.0 -p 4567
We specify the host the application will run on as well as the port it will listen on in this command.
Run the Sinatra Application
Let’s build the docker image once more now that we have added these additional lines to the Dockerfile.

The docker image should successfully build at this point. Now we can spin up a container based on that image.
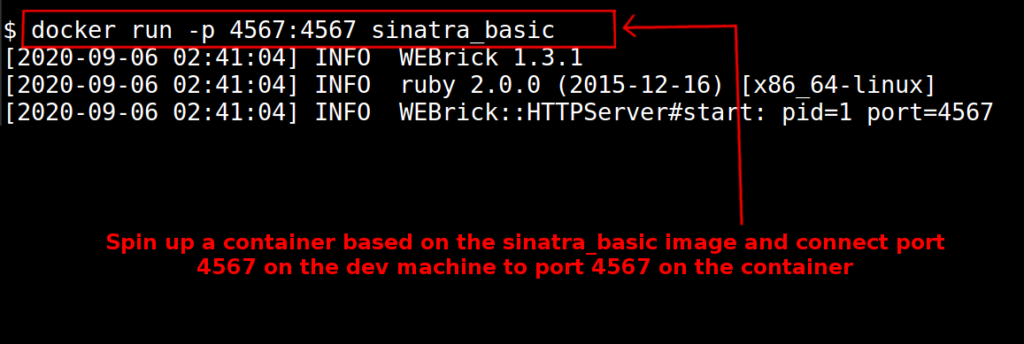
As you can see from the Terminal, it looks like the application is up and running. Let’s send a request to the application using cURL.
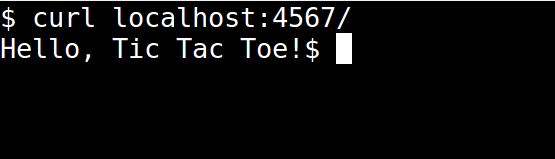
Success! We made a request to the /
endpoint for our application and got the response “Hello, Tic Tac Toe!” Now we can check off the last remaining items on our definition of done list:
- Start up a basic Sinatra application in a Docker container, then successfully hit the
/
route. [DONE]The application should run on Ruby 2.0.0[DONE]Hitting[DONE]/
should return a response with the text “Hello, Tic Tac Toe!”
Conclusion
Now that we have verified that we can run a basic Sinatra application in a Docker container on Ruby 2.0.0, we are on our way. We kept the surface area of concern small by focusing first on containerizing a simple Ruby 2.0.0 application. Then we focused on containerizing a simple Sinatra application.
We learned that we needed to explicitly specify a version for the Sinatra gem to keep all of its dependencies compatible with the old version of Ruby.
In Part 2 of Code Reading #1, we will begin looking at layering the test suite back in.